mirror of
https://github.com/verdaccio/verdaccio.git
synced 2024-11-13 03:35:52 +01:00
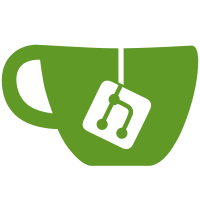
* feat: add support for profile cli command #392 - it allows to update password npm profile set password - display current profile npm profile get https://docs.npmjs.com/cli/profile * chore: update @verdaccio/types@4.0.0 * feat: add min password length on npm by defaul is min 7 characters, this might be configurable in the future. * chore: update verdaccio-htpasswd@1.0.1 * refactor: update unit test * refactor: provide friendly error for tfa request * test: api profile unit test * chore: fix eslint comment * test: update profile test * chore: set mim as 3 characters
102 lines
2.4 KiB
JavaScript
102 lines
2.4 KiB
JavaScript
/**
|
|
* @prettier
|
|
*/
|
|
|
|
// @flow
|
|
|
|
// this file is not aim to be tested, just to check flow definitions
|
|
|
|
import Config from '../../../../src/lib/config';
|
|
import LoggerApi from '../../../../src/lib/logger';
|
|
|
|
import type { Config as AppConfig, PackageAccess, IPluginAuth, RemoteUser, Logger, PluginOptions } from '@verdaccio/types';
|
|
|
|
class ExampleAuthPlugin implements IPluginAuth {
|
|
config: AppConfig;
|
|
logger: Logger;
|
|
|
|
constructor(config: AppConfig, options: PluginOptions) {
|
|
this.config = config;
|
|
this.logger = options.logger;
|
|
}
|
|
|
|
adduser(user: string, password: string, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
changePassword(username, password, newPassword, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
authenticate(user: string, password: string, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
allow_access(user: RemoteUser, pkg: PackageAccess, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
allow_publish(user: RemoteUser, pkg: PackageAccess, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
}
|
|
|
|
type SubTypePackageAccess = PackageAccess & {
|
|
sub?: boolean,
|
|
};
|
|
|
|
class ExampleAuthCustomPlugin implements IPluginAuth {
|
|
config: AppConfig;
|
|
logger: Logger;
|
|
|
|
constructor(config: AppConfig, options: PluginOptions) {
|
|
this.config = config;
|
|
this.logger = options.logger;
|
|
}
|
|
|
|
adduser(user: string, password: string, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
changePassword(username, password, newPassword, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
authenticate(user: string, password: string, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
allow_access(user: RemoteUser, pkg: SubTypePackageAccess, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
|
|
allow_publish(user: RemoteUser, pkg: SubTypePackageAccess, cb: verdaccio$Callback): void {
|
|
cb();
|
|
}
|
|
}
|
|
|
|
const config1: AppConfig = new Config({
|
|
storage: './storage',
|
|
self_path: '/home/sotrage',
|
|
});
|
|
|
|
const options: PluginOptions = {
|
|
config: config1,
|
|
logger: LoggerApi.logger.child(),
|
|
};
|
|
|
|
const auth = new ExampleAuthPlugin(config1, options);
|
|
const authSub = new ExampleAuthCustomPlugin(config1, options);
|
|
const remoteUser: RemoteUser = {
|
|
groups: [],
|
|
real_groups: [],
|
|
name: 'test',
|
|
};
|
|
|
|
auth.authenticate('user', 'pass', () => {});
|
|
auth.allow_access(remoteUser, {}, () => {});
|
|
auth.allow_publish(remoteUser, {}, () => {});
|
|
authSub.authenticate('user', 'pass', () => {});
|
|
authSub.allow_access(remoteUser, { sub: true }, () => {});
|
|
authSub.allow_publish(remoteUser, { sub: true }, () => {});
|