mirror of
https://github.com/verdaccio/verdaccio.git
synced 2024-11-13 03:35:52 +01:00
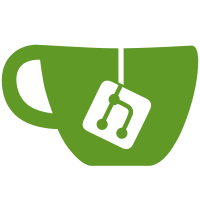
* feat: flexible template generator and manifest * chore: add changeset * chore: restore dep * chore: add docs * chore: update snapshots * chore: update docker examples for v5 * chore: refactor web module * chore: format * chore: refactor web api endpoints * test: add test for user login web * chore: refactor endpoints * chore: fix merge * chore: fix merge * Update ci.yml * chore: test * chore: add static * chore: update script * chore: fix e2e * chore: fix method * docs: update v5 relative docker example * chore: update html render * chore: update style * Update .prettierignore * chore: update changeset * chore: use pnpm6 on run test temporary ci * chore: drop node 16 for pnpm 6 * chore: update ci * chore: update ci * chore: update ci * chore: update ci * chore: remove circle ci * chore: better url prefix handling * chore: format code * chore: remove test node 10 * docs: add docker v5 relative revers proxy example * chore: use base html tag * chore: update test
62 lines
1.7 KiB
TypeScript
62 lines
1.7 KiB
TypeScript
import semver from 'semver';
|
|
import { addNpmPrefix, addRegistry, addYarnPrefix, initialSetup, Setup } from '../utils/registry';
|
|
import { npm, pnpm, yarn, yarnWithCwd } from '../utils/process';
|
|
import { createProject } from '../utils/utils';
|
|
|
|
describe('install a package', () => {
|
|
jest.setTimeout(90000);
|
|
const port = '9011';
|
|
let setup: Setup;
|
|
|
|
beforeAll(async () => {
|
|
setup = await initialSetup(port);
|
|
});
|
|
|
|
test('should run npm install', async () => {
|
|
const projectFolder = createProject('webpack-npm-jest');
|
|
const resp = await npm(
|
|
'install',
|
|
'jest',
|
|
'--json',
|
|
...addNpmPrefix(projectFolder),
|
|
...addRegistry(port)
|
|
);
|
|
expect(resp.stderr).toBeUndefined();
|
|
|
|
const resp2 = await npm('run', 'jest', '--version', ...addNpmPrefix(projectFolder));
|
|
expect(semver.valid(resp2.stdout)).toBeTruthy();
|
|
});
|
|
|
|
test('should run pnpm install', async () => {
|
|
const projectFolder = createProject('webpack-pnpm-test');
|
|
const resp = await pnpm(
|
|
'install',
|
|
'jest@26.6.3',
|
|
...addNpmPrefix(projectFolder),
|
|
...addRegistry(port)
|
|
);
|
|
expect(resp.stderr).toBeUndefined();
|
|
|
|
// TODO: verify package was correctly installed
|
|
});
|
|
|
|
test('should run yarn classic install', async () => {
|
|
const projectFolder = createProject('jest-yarn-test');
|
|
const resp = await yarn(
|
|
'add',
|
|
'jest@26.6.3',
|
|
...addYarnPrefix(projectFolder),
|
|
...addRegistry(port)
|
|
);
|
|
expect(resp.stderr).toBeUndefined();
|
|
|
|
const resp2 = await yarnWithCwd(projectFolder, 'jest', '--version');
|
|
// yarn output is to verbose
|
|
expect(resp2.stdout).toMatch(/26.6.3/);
|
|
});
|
|
|
|
afterAll(async () => {
|
|
setup.child.kill();
|
|
});
|
|
});
|