mirror of
https://github.com/verdaccio/verdaccio.git
synced 2024-11-13 03:35:52 +01:00
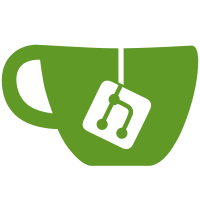
* #2606 add prettier plugin sort imprts * #2606 update pnpm-lock.yaml * #2606 update eslint rules * #2606 fixes website directory formatting Co-authored-by: Ayush Sharma <ayush.sharma@trivago.com>
65 lines
1.5 KiB
TypeScript
65 lines
1.5 KiB
TypeScript
import express from 'express';
|
|
import path from 'path';
|
|
import request from 'request';
|
|
|
|
import { parseConfigFile } from '@verdaccio/config';
|
|
import { API_ERROR } from '@verdaccio/core';
|
|
import { setup } from '@verdaccio/logger';
|
|
|
|
import endPointAPI from '../../src';
|
|
|
|
setup([{ type: 'stdout', format: 'pretty', level: 'trace' }]);
|
|
|
|
const app = express();
|
|
const server = require('http').createServer(app);
|
|
|
|
const parseConfigurationFile = (conf) => {
|
|
return path.join(__dirname, `./${conf}`);
|
|
};
|
|
|
|
// TODO: restore when web module is ready
|
|
describe.skip('basic system test', () => {
|
|
let port;
|
|
jest.setTimeout(20000);
|
|
|
|
beforeAll(async function (done) {
|
|
const config = parseConfigFile(parseConfigurationFile('basic.yaml'));
|
|
app.use(await endPointAPI(config));
|
|
server.listen(0, function () {
|
|
port = server.address().port;
|
|
done();
|
|
});
|
|
});
|
|
|
|
afterAll((done) => {
|
|
server.close(done);
|
|
});
|
|
|
|
test('server should respond on /', (done) => {
|
|
request(
|
|
{
|
|
url: 'http://localhost:' + port + '/',
|
|
},
|
|
function (err, res, body) {
|
|
expect(err).toBeNull();
|
|
expect(body).toMatch(/Verdaccio/);
|
|
done();
|
|
}
|
|
);
|
|
});
|
|
|
|
test('server should respond on /___not_found_package', (done) => {
|
|
request(
|
|
{
|
|
json: true,
|
|
url: `http://localhost:${port}/___not_found_package`,
|
|
},
|
|
function (err, res, body) {
|
|
expect(err).toBeNull();
|
|
expect(body.error).toMatch(API_ERROR.NO_PACKAGE);
|
|
done();
|
|
}
|
|
);
|
|
});
|
|
});
|