mirror of
https://github.com/excalidraw/excalidraw.git
synced 2024-11-10 11:35:52 +01:00
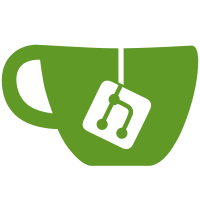
* fix: Enable jsx transform in webpack
* update changelog
* fix
* typo fix in script
* docs: release @excalidraw/excalidraw@0.9.1 🎉
* fix changelog
* release 0.10.0
* Update src/packages/excalidraw/CHANGELOG.md
40 lines
1.1 KiB
JavaScript
40 lines
1.1 KiB
JavaScript
const fs = require("fs");
|
|
const util = require("util");
|
|
const exec = util.promisify(require("child_process").exec);
|
|
const updateReadme = require("./updateReadme");
|
|
const updateChangelog = require("./updateChangelog");
|
|
|
|
const excalidrawDir = `${__dirname}/../src/packages/excalidraw`;
|
|
const excalidrawPackage = `${excalidrawDir}/package.json`;
|
|
|
|
const updatePackageVersion = (nextVersion) => {
|
|
const pkg = require(excalidrawPackage);
|
|
pkg.version = nextVersion;
|
|
const content = `${JSON.stringify(pkg, null, 2)}\n`;
|
|
fs.writeFileSync(excalidrawPackage, content, "utf-8");
|
|
};
|
|
|
|
const release = async (nextVersion) => {
|
|
try {
|
|
updateReadme();
|
|
await updateChangelog(nextVersion);
|
|
updatePackageVersion(nextVersion);
|
|
await exec(`git add -u`);
|
|
await exec(
|
|
`git commit -m "docs: release @excalidraw/excalidraw@${nextVersion} 🎉"`,
|
|
);
|
|
/* eslint-disable no-console */
|
|
console.log("Done!");
|
|
} catch (e) {
|
|
console.error(e);
|
|
process.exit(1);
|
|
}
|
|
};
|
|
|
|
const nextVersion = process.argv.slice(2)[0];
|
|
if (!nextVersion) {
|
|
console.error("Pass the next version to release!");
|
|
process.exit(1);
|
|
}
|
|
release(nextVersion);
|